Sending Zoho emails via SMTP using PHP Swiftmailer
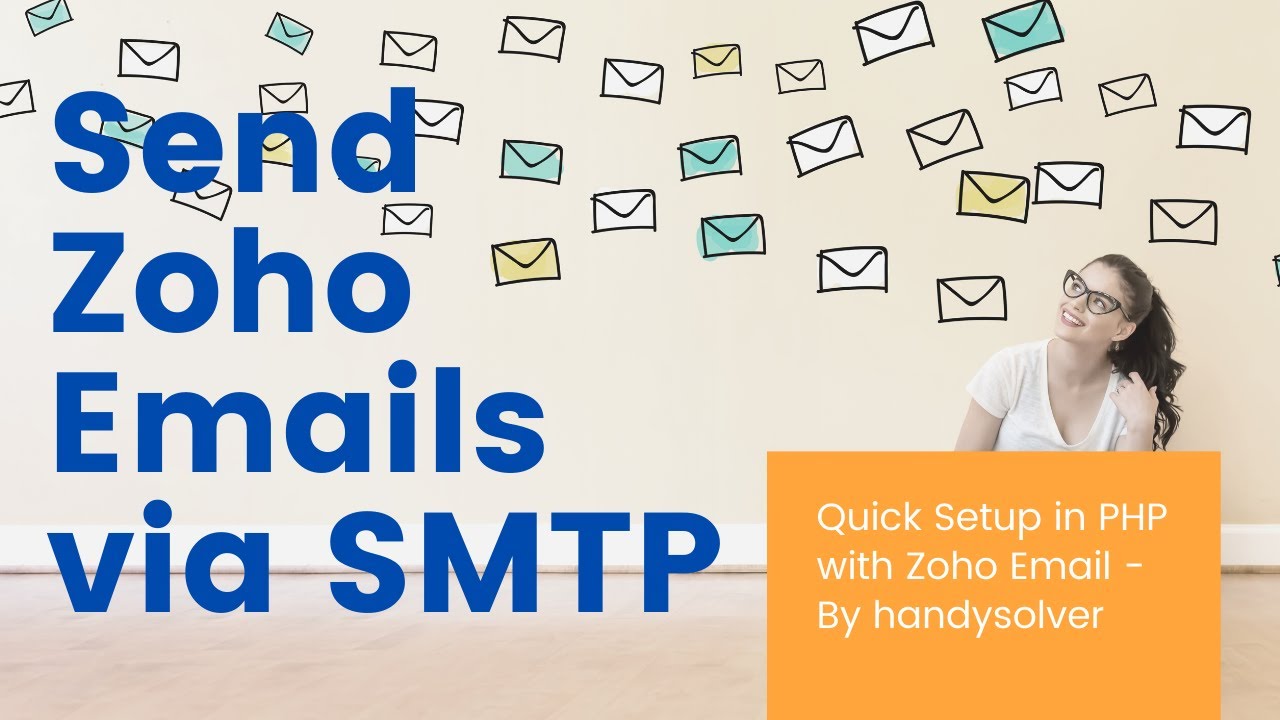
The aim of this tutorial is to quickly show how Zoho Email can be used in your PHP code to send emails.
There is also a quick video explaining this:
The code is as follows:
class ZohoEmail { public $toEmail = '[email protected]'; public $subject = 'Test subject'; public $body = 'Test message'; public $host = 'smtp.zoho.in';//Country specific public $port = '587'; public $encryption = 'tls'; public $username = '[email protected]'; public $password = 'YourZohoPassword'; public $fromName = 'Your Name'; public $fromEmail = '[email protected]'; public function actionEmail(){ $transport = (new Swift_SmtpTransport($this->host, $this->port)) ->setUsername($this->username) ->setPassword($this->password) ->setEncryption($this->encryption); $mailer = new Swift_Mailer($transport); $message = (new Swift_Message($this->subject)) ->setFrom([$this->fromEmail => $this->fromName]) ->setTo($this->toEmail) ->setBody($this->body, 'text/html'); $mailer->send($message); } }
Documentation that helped
https://www.zoho.com/mail/help/zoho-smtp.html
The forum thread regarding the correct SMTP Server
https://help.zoho.com/portal/en/community/topic/535-authentication-failed-code-535
Special cases (2 Factor Authentication on)
https://www.zoho.com/mail/help/zoho-smtp.html
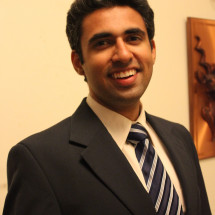
Rahul Matharu